How to Implement TreeMap in Java
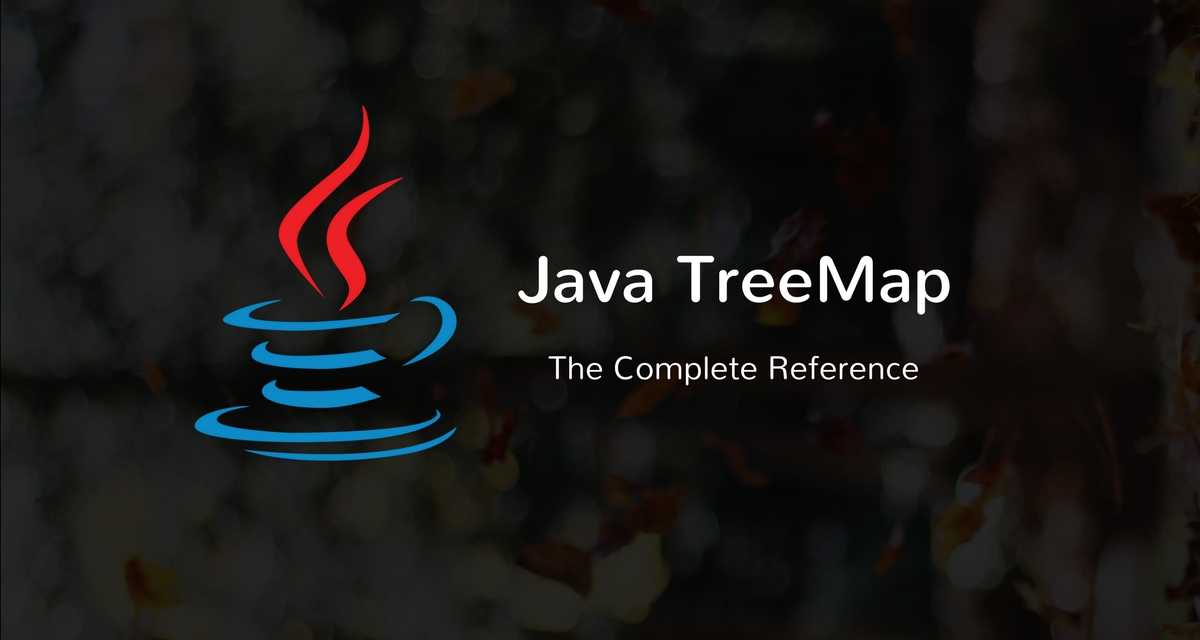
TreeMap is a widely used implementation of the Map interface in Java. It is based on a red-black tree, which provides a balanced and ordered data structure. TreeMap offers many features, such as sorting, searching, and traversal, making it a popular choice for many Java developers. In this blog post, we will discuss how to implement TreeMap in Java.
Step 1:
Import the TreeMap Class To use TreeMap in Java, you must first import the TreeMap class from the java.util package. You can do this by adding the following line of code at the top of your Java file:
import java.util.TreeMap;
Step 2:
Create a TreeMap Object After importing the TreeMap class, you can create a TreeMap object by instantiating it with the new keyword:
TreeMap<String, Integer> treeMap = new TreeMap<>();
This creates a new empty TreeMap with key and value types String and Integer, respectively. You can replace these types with any other types that you need.
Step 3:
Add Elements to the TreeMap To add elements to the TreeMap, you can use the put() method. The put() method takes two arguments: the key and the value. The key is used to index the value in the TreeMap, and it must be unique. The value is the data associated with the key. Here’s an example:
treeMap.put("apple", 10);
treeMap.put("banana", 20);
treeMap.put("orange", 30);
This adds three key-value pairs to the TreeMap, with keys “apple”, “banana”, and “orange”, and values 10, 20, and 30, respectively.
Step 4:
Retrieve Elements from the TreeMap To retrieve elements from the TreeMap, you can use the get() method. The get() method takes one argument: the key of the element you want to retrieve. Here’s an example:
int value = treeMap.get("banana");
System.out.println("Value of banana: " + value);
This retrieves the value associated with the key “banana” from the TreeMap and prints it to the console.
Step 5:
Remove Elements from the TreeMap To remove elements from the TreeMap, you can use the remove() method. The remove() method takes one argument: the key of the element you want to remove. Here’s an example:
treeMap.remove("orange");
This removes the key-value pair with the key “orange” from the TreeMap.
Step 6:
Traverse the TreeMap To traverse the elements of the TreeMap, you can use various methods such as keySet(), values(), and entrySet(). Here’s an example:
for (Map.Entry<String, Integer> entry : treeMap.entrySet()) {
System.out.println("Key: " + entry.getKey() + " Value: " + entry.getValue());
}
This loops through each key-value pair in the TreeMap and prints them to the console.
In conclusion, TreeMap is a powerful implementation of the Map interface in Java. With its ordered and balanced data structure, it offers many features that can be useful in a variety of situations. By following the steps outlined in this blog post, you can easily implement TreeMap in your Java programs.